This post explains how to make your own timtable display. In principle it is a Raspberry Pi running a Python program which is connected to a monitor. It can be useful to have a display showing public transport connections close to your office for example. Follow this to make your own display table.
Requirements
Timetable Python Code
We are going to use an (inofficial) API by fahrplan.guru . This site is intended for german public transport but it works for other european cities like Vienna, Paris or Rome too. You can do a quick check on their website to see if they have information about your city.
We are going to use this site's option to show all outgoing connections from a specific station.
1) Retrieve Information For Fome Station (E.g. Berlin Hauptbahnhof)
Import libraries
import requests
import json
Define function for each stop you want to add
def times_berlin_hbf(time, date, timetable):
Our function takes three parameters. 'time' and 'date' contain the current time and date so that we can make a request for the desired departing time. 'timetable' is a list where each index indicates a stop.
Retrieve information from API
url = 'https://www.fahrplan.guru/api/haltestelle?city=berlin&date=' + date + '&dir=dep&name=berlin-hauptbahnhof&state=berlin&time=' + time
res = requests.get(url)
res = json.loads(res.text)
We resolve our request as json to be able to easily extract the information we want.
Add the stop to timetable
timetable.append(0)
In our case 0 indicates Berlin Hauptbahnhof. Each stop you add should have a unique id
Extract desired information
for i in range(5):
berlin_hbf_entry = []
berlin_hbf_entry.append(res["transports"][i]["nearest_trip_time"])
berlin_hbf_entry.append(res["transports"][i]["transport_info"]["full_name"])
berlin_hbf_entry.append(res["transports"][i]["stop_title"])
timetable.append(berlin_hbf_entry)
We create another list where we store our extracted information. We want the departure time, the bus/train/metro line and its destination. You can inspect the json file and extract further information that you find useful.
2) Display Extracted Information
Import libraries
import os
import datetime
from time import sleep
from stops.berlin_hbf import times_berlin_hbf
Import the stop we created in the previous step.
Get current date
def get_date():
today = datetime.date.today()
day = datetime.datetime.strptime(str(today.day), "%d").strftime("%d")
month = datetime.datetime.strptime(str(today.month), "%m").strftime("%m")
year = datetime.datetime.strptime(str(today.year), "%Y").strftime("%Y")
return day + '.' + month + '.' + year
Get current time
def get_time(station):
now = datetime.datetime.now()
if station == 'berlin_hbf':
now + datetime.timedelta(minutes=2)
elif station == 'berlin_pankow':
now + datetime.timedelta(minutes=3)
hour = datetime.datetime.strptime(str(now.hour), "%H").strftime("%H")
minute = datetime.datetime.strptime(str(now.minute), "%M").strftime("%M")
return hour + '%3A' + minute
Add a few extra minutes to the current time to only show the connections that are reachable in time. You can adjust the value for each stop depending on how long it takes to get there.
Format and print timetable
def get_timetable(timetable):
times_berlin_hbf(get_time('berlin_hbf'), get_date(), timetable)
times_berlin_pankow(get_time('berlin_pankow'), get_date(), timetable)
for row in timetable:
if row == 0:
print('\nBERLIN HAUPTBAHNHOF')
continue
if row == 1:
print('\nBERLIN-PANKOW')
continue
print({: >20} {: >20} {: >50}.format(*row))
First Retrieve stops we want to display which get added to timetable. Then for each stop in timetable print the matching stop name and add some spacing between the departure time, line and destination so it is easier to read.
Call the timetable function
while True:
timetable = []
_ = os.system('clear')
get_timetable(timetable)
sleep(30)
We clear the terminal every time before we print the timetable. Then we call the timetable and wait 30 seconds before we reapeat this process.
You can check out the full source code on
3) Configure Raspberry Pi
Install Raspberry Pi OS
Run startup script on Pi
#!/usr/bin/env bash
lxterminal --command="/usr/bin/python /home/notadmin/Documents/kvv-timetable/main.py"
Create a bash script 'autostart-timetable' and make it executable by running chmod +x autostart-timetable
.
Now make the script run automatically every time the Pi is booted by adding @path/to/autostart-timetable
to the LXDE autostart file. I stored 'autostart-timetable' in ~/Documents/
and useed a user specific LXDE autostart file so I added @Documents/autostart-timetable
to~/.config/lxsession/LXDE-pi/autostart
.
Here is a more detailed explanation about autostart on Pi.
4) End
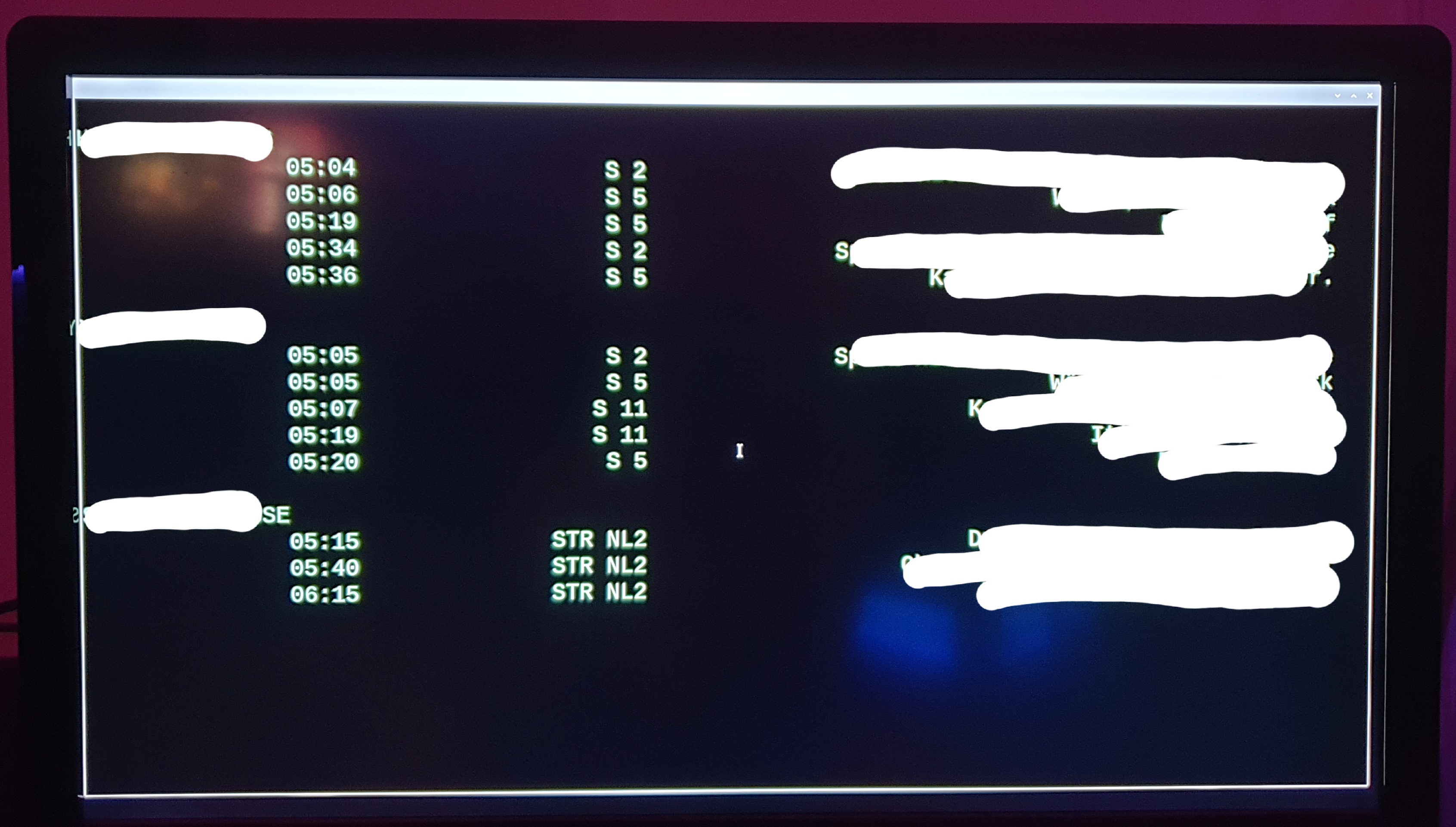